A Guide to Python Databases in Web Applications
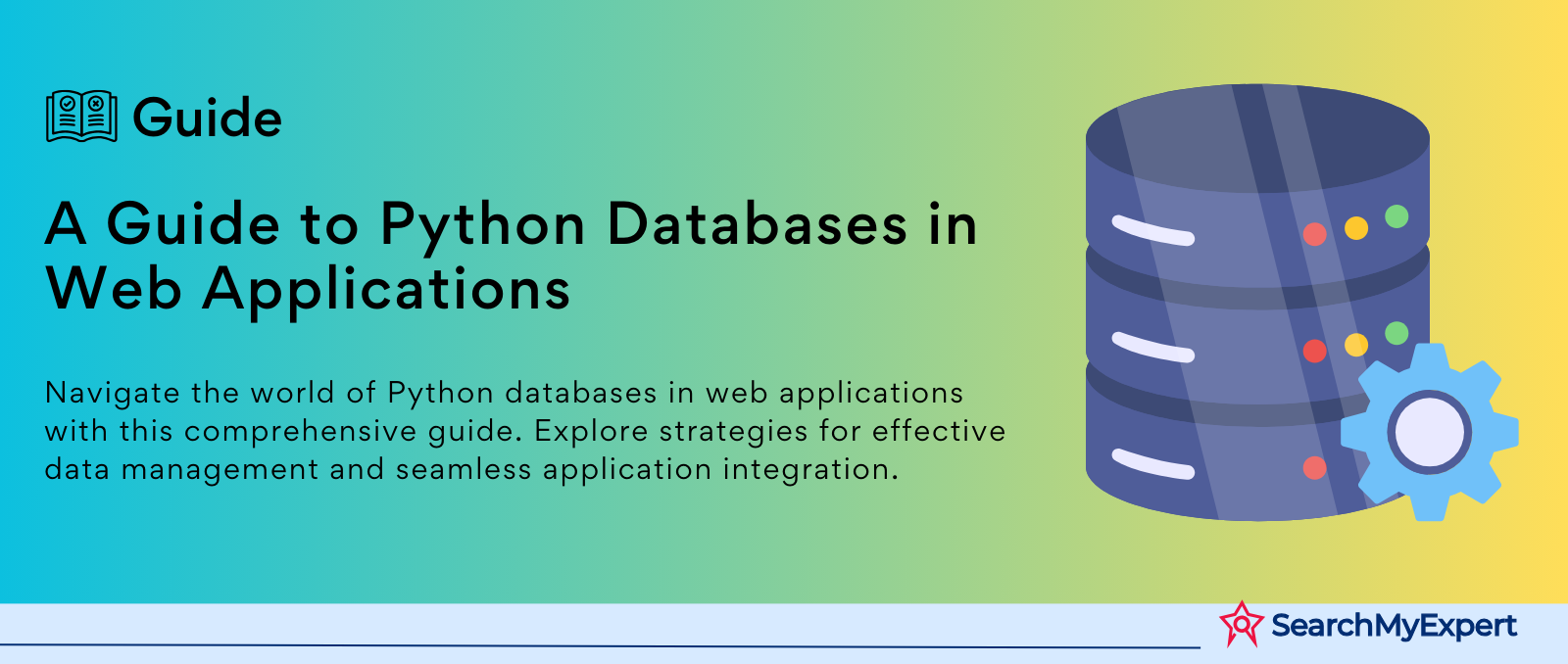
Database Integration in Web Applications with Python
In today's digital age, web applications are more than just online platforms; they are intricate ecosystems that manage, process, and display data in real time. At the heart of these dynamic web applications lies the crucial role of databases. They are the repositories where data is stored, organized, and retrieved. Integrating databases with web applications is not just a technical necessity; it’s a strategic approach to enhance functionality, user experience, and overall performance.
Understanding Web Applications and Databases
Web applications, unlike static websites, are interactive platforms that allow users to create, manipulate, and interact with data stored on a server. A typical web application architecture involves a front-end (client side), where the user interacts, and a back-end (server side), which processes the client’s requests, manages data, and communicates with the database. The database, in this structure, is the cornerstone, acting as the central repository where all data is stored and managed.
The Imperative of Database Integration
In the realm of web development, the integration of databases with web applications is not just an option; it's a necessity. This integration allows for dynamic content generation, user authentication, data analysis, and real-time data updates. For instance, when you shop online, it's the database integration that helps the application remember your preferences, suggest relevant products, and store your shopping history.
Why Python for Database Integration?
Python, known for its simplicity and readability, is a favorite among developers for web application development. Here’s why Python stands out for database integration:
- Ease of Use: Python’s syntax is clear and intuitive, making it easier for developers to write and maintain code that interacts with databases.
- Rich Set of Libraries: Python offers a plethora of libraries like SQLAlchemy, Django ORM, and Peewee, which simplify database interactions and abstract many of the complexities.
- Versatility: Python supports multiple database engines, including MySQL, PostgreSQL, and SQLite, offering flexibility to work with various database systems.
- Community and Support: Python’s large community means extensive support, tutorials, and shared knowledge, which is invaluable for troubleshooting and learning.
Choosing the Right Database Technology for Python Web Applications
Selecting the appropriate database technology is a pivotal decision in the development of a web application. This choice directly impacts the application's performance, scalability, and maintenance. Python, being versatile, supports a wide range of databases, each with its unique features and capabilities. Understanding these options and knowing what to consider when choosing a database is crucial for the success of your web application.
Popular Database Options for Python Web Apps
- MySQL: A widely-used open-source relational database, MySQL is known for its reliability and ease of use. It is a go-to choice for applications that require a robust, transactional database with a rich ecosystem of tools and community support.
- PostgreSQL: Another powerful open-source relational database, PostgreSQL is renowned for its advanced features, such as support for complex queries, concurrency without read locks, and extensibility. It's ideal for applications that require sophisticated data manipulation and analytics capabilities.
- MongoDB: Stepping into the realm of NoSQL databases, MongoDB offers a flexible, schema-less model that allows you to store documents in a JSON-like format. This database is suitable for applications that require rapid development and handling of diverse data types.
Factors to Consider When Choosing a Database
- Project Requirements: Understand the nature of your application. Does it require complex transactions, or is it read-heavy? For transactional requirements, relational databases like MySQL or PostgreSQL are preferable, while MongoDB might be better for applications with unstructured data.
- Data Size and Scalability: Consider the amount of data your application will handle. For large datasets or applications that need to scale dynamically, NoSQL databases like MongoDB offer advantages.
- Performance Needs: Different databases have different strengths in terms of performance. While relational databases offer robust transaction management, NoSQL databases can provide faster read/write capabilities for certain types of data.
- Community Support and Ecosystem: Look for a database with strong community support and a rich ecosystem of tools. This is crucial for finding resources, tutorials, and third-party tools to assist in development and problem-solving.
Introduction to Object-Relational Mappers (ORMs)
An Object-Relational Mapper (ORM) is a code library that automates the transfer of data stored in relational databases into objects that are more commonly used in application code. ORMs are beneficial because they:
- Simplify Code: Instead of writing SQL queries, developers interact with the database using the programming language they are working with, in this case, Python.
- Improve Code Maintainability: ORMs help in keeping the database access code neat, organized, and more maintainable.
- Enhance Portability: With ORMs, it's easier to switch between different database technologies as the ORM abstracts the database-specific details.
Establishing Database Connection in Python Web Applications
For a Python web application, establishing a database connection is a critical step. This involves not only the technical aspect of connecting to the database but also ensuring effective management of these connections for optimal performance. Understanding connection pools, configuring database settings, and utilizing Python libraries for connectivity are essential components of this process.
Understanding Connection Pools and Connection Management
- Connection Pool Basics: A connection pool is a collection of database connections that are kept open and reused for future requests. This approach is more efficient than opening and closing a connection for each interaction with the database.
- Benefits of Connection Pools: They enhance performance by reducing the overhead associated with establishing a connection. Additionally, they help in managing the number of connections, which is crucial for resource management and scalability.
- Effective Connection Management: Proper management of connection pools involves handling the lifecycle of connections, such as opening, closing, and monitoring their health. This ensures a stable and efficient database interaction environment.
Setting Up Database Configuration Files and Environment Variables
- Utilizing Configuration Files: Storing database configurations (like URLs, usernames, and passwords) in separate configuration files is a best practice. It keeps the code clean and makes it easier to update settings without modifying the codebase.
- Securing Sensitive Data with Environment Variables: Environment variables are key for securing sensitive information such as database passwords. By setting these variables outside of the application, they can be accessed securely within the code, providing an additional layer of security.
Connecting to the Database Using Python Libraries
- Choosing the Right Library: Python offers several libraries for database connectivity, with SQLAlchemy being a popular choice. These libraries provide a high-level abstraction for database interaction, simplifying the connection process.
- Connection Pooling with Python Libraries: Libraries like SQLAlchemy come with built-in support for connection pooling. They manage the creation and lifecycle of connections, ensuring efficient use of resources.
- Leveraging Library Features for Efficient Connectivity: When using a library like SQLAlchemy, you can take advantage of its features to manage connections effectively. This includes configuring pool sizes, managing timeouts, and handling disconnections gracefully.
Performing CRUD Operations in Python Web Applications
CRUD operations – Create, Read, Update, and Delete – are the four basic functions of persistent storage in web applications. Mastering these operations is essential for manipulating and managing data effectively. In Python web applications, these operations can be performed neatly and efficiently using Object-Relational Mapping (ORM) methods. Additionally, handling errors and exceptions is crucial to ensure the robustness of the application.
Understanding CRUD Operations
- Create: This operation involves adding new records to the database. It's the starting point of data entry into your application.
- Read: Reading refers to fetching data from the database. This can range from simple queries fetching specific records to complex ones involving joins and filters.
- Update: Updating is the process of modifying existing data in the database. This could be changing a user's details, updating a product's price, etc.
- Delete: Delete operations remove records from the database. It is crucial to handle delete operations carefully to avoid unintended data loss.
Executing CRUD Operations Using ORM Methods
- Using ORM for CRUD: ORMs in Python, such as SQLAlchemy or Django ORM, provide an intuitive way to perform CRUD operations without writing raw SQL queries. These methods abstract the database layer, allowing developers to interact with the database using Python objects and methods.
- Benefits of ORM Methods: They simplify database interactions, make the code more readable, and reduce the likelihood of SQL injection attacks. ORM methods also help in maintaining database agnosticism, making the code more portable.
Handling Errors and Exceptions During Database Interactions
- Importance of Error Handling: Proper error handling ensures that your application can gracefully handle unexpected situations, like database connection failures, query errors, or data integrity issues.
- Common Database Errors: These include connection errors, data integrity errors, query syntax errors, and timeout errors. Each of these errors should be handled appropriately to maintain the stability of the application.
- Implementing Try-Except Blocks: Python’s try-except blocks can be used to catch and handle exceptions. This allows you to provide user-friendly error messages and, if necessary, roll back changes to maintain data consistency.
- Logging: Logging errors is vital for diagnosing issues in production. Detailed logs help in quickly identifying and fixing problems, enhancing the reliability of the application.
Implementing Data Validation and Security in Python Web Applications
In the digital era, where data is a valuable asset, ensuring the integrity and security of this data is paramount. Implementing robust data validation and security measures in Python web applications is not just a best practice; it’s a necessity. This step involves thorough validation of user input, sanitization to prevent security breaches, and implementing authentication and authorization mechanisms for secure access.
Understanding the Importance of Data Validation and User Input Sanitization
- Data Validation: This is the process of ensuring that user inputs meet the application's requirements and constraints before being processed or stored in the database. Effective data validation minimizes the chances of corrupt or erroneous data entering the system.
- User Input Sanitization: Input sanitization is crucial for security. It involves cleaning user input to prevent malicious data, such as SQL injection or cross-site scripting (XSS) attacks, from exploiting the application.
Techniques for Validating User Input
- Data Type Validation: Ensure that the input matches the expected data type (e.g., string, integer, date).
- Length Restrictions: Implement length checks to prevent overly long inputs that could cause performance issues or buffer overflows.
- Format Validation: For certain types of data, like email addresses or phone numbers, validate the format to ensure data consistency and reliability.
- Range Checking: For numerical inputs, ensure values fall within a defined range.
- Regular Expressions: Utilize regular expressions for complex validations, such as password strength requirements.
Implementing User Authentication and Authorization Mechanisms
- User Authentication: Authentication is the process of verifying the identity of a user. This usually involves checking credentials, like a username and password, against stored values in the database.
- Secure Password Handling: Implement secure practices for password handling, such as hashing passwords before storing them and using salts to prevent rainbow table attacks.
- Session Management: Properly manage user sessions to ensure that only authenticated users can access their accounts and session data is protected.
- User Authorization: Authorization involves determining if an authenticated user has the right to perform certain actions or access specific resources within the application. This can be managed through roles, permissions, or access control lists.
- Implementing Security Protocols: Utilize security protocols and best practices, such as HTTPS, to encrypt data in transit and protect against interception or eavesdropping.
Optimizing Database Performance in Python Web Applications
Database performance optimization is a key aspect of efficient web application development. It involves identifying and mitigating common bottlenecks, refining queries and schema design, and implementing caching strategies for frequently accessed data. By optimizing database interactions, you can significantly enhance the overall performance and user experience of your Python web application.
Understanding Common Database Performance Bottlenecks
- Inefficient Queries: Queries that are poorly written or overly complex can significantly slow down database performance.
- Poorly Designed Schema: A database schema that is not optimized for the queries being run can lead to unnecessary data processing and slow response times.
- Lack of Indexes: Without proper indexing, the database has to scan the entire table to find relevant data, which can be time-consuming.
- Hardware Limitations: Insufficient memory, CPU power, or disk space can also contribute to performance issues.
- Concurrency and Locking Issues: In situations where multiple processes are accessing or modifying data concurrently, improper management can lead to locking and blocking, affecting performance.
Techniques for Optimizing Queries and Schema Design
- Query Optimization: Simplify and optimize SQL queries for efficiency. This may include selecting only necessary fields, avoiding unnecessary joins, and using subqueries judiciously.
- Effective Indexing: Implement indexes on columns that are frequently used in WHERE clauses, JOIN conditions, or as SORT keys. However, be cautious with indexing as over-indexing can slow down write operations.
- Schema Refinement: Design the database schema to align with the application’s access patterns. Normalize the schema to eliminate redundancy but also consider denormalization in certain scenarios for quicker reads.
- Using Query Profiling Tools: Utilize tools to analyze and understand how queries are executed. This can help in identifying slow queries and the reasons behind their performance issues.
Utilizing Caching Mechanisms for Frequently Accessed Data
- Caching Basics: Caching involves temporarily storing copies of frequently accessed data in a faster storage system. This significantly reduces the number of times the application needs to query the database, thereby improving performance.
- Implementing Application-Level Caching: Use caching mechanisms like Memcached or Redis. These systems can cache query results, computed data, or session information, reducing database load.
- Database-Level Caching: Most modern databases come with built-in caching capabilities. Ensuring these are properly configured can lead to significant performance improvements.
- Cache Invalidation: Implement a strategy to update or invalidate the cache when the underlying data changes to ensure that users always see up-to-date information.
Deployment and Maintenance of Database-Driven Web Applications
Deploying and maintaining a database-driven web application requires careful planning and ongoing management to ensure its reliability, performance, and security. This involves considerations for the deployment environment, monitoring database activity, and implementing strategies for backups and disaster recovery. By addressing these areas, you can establish a robust framework for your application's lifecycle.
Considerations for Deploying Database-Driven Web Applications
- Choosing the Right Hosting Environment: Select a hosting solution that matches your application's requirements in terms of scalability, security, and performance. Cloud services like AWS, Google Cloud, and Azure offer scalable infrastructure that can adapt to your application's needs.
- Configuration Management: Ensure that your database and application configurations are properly set for the production environment. This includes setting up environment variables for sensitive information and configuring database connections securely.
- Security Measures: Implement security best practices, such as securing connections with SSL/TLS, regularly updating software to patch vulnerabilities, and adhering to the principle of least privilege for database access.
Implementing Logging and Monitoring for Database Activity
- Logging: Set up comprehensive logging for your application and database. Logs should capture errors, transactions, and suspicious activities to aid in debugging and monitoring security.
- Monitoring: Use tools to monitor database performance and health in real-time. Monitoring can help identify bottlenecks, track resource usage, and alert you to potential issues before they become critical.
- Analysis Tools: Utilize tools for log analysis and performance tracking. These can provide insights into usage patterns, slow queries, and system performance, helping you make informed optimization decisions.
Strategies for Database Backups and Disaster Recovery
- Regular Backups: Implement a schedule for regular database backups. This includes full backups as well as incremental backups to minimize data loss and ensure that you can restore the database to a specific point in time if needed.
- Backup Storage: Store backups in a secure, off-site location. Using cloud storage solutions can provide scalability and reliability for storing backup data.
- Disaster Recovery Planning: Develop a comprehensive disaster recovery plan that outlines procedures for restoring data and services in the event of a system failure or data loss. This plan should include details on backup restoration, roles and responsibilities, and communication strategies.
- Testing Recovery Procedures: Regularly test your backup and recovery procedures to ensure they are effective and that your team is familiar with the process. This helps to minimize downtime and data loss during an actual disaster.
Conclusion
Integrating databases into Python web applications encompasses a broad range of practices, from choosing the right database technology and establishing secure connections to optimizing database performance and ensuring robust deployment and maintenance strategies. Throughout this guide, we've explored the essentials of database integration, including the advantages of using Python, performing CRUD operations, implementing data validation and security measures, and optimizing database interactions for scalability and efficiency.
The deployment and ongoing maintenance of database-driven applications require careful consideration to security, logging and monitoring, and disaster recovery plans. By adhering to the strategies and best practices outlined, developers can create dynamic, efficient, and secure web applications that stand the test of time.
Enhance your IT projects with
Python Development Service Companies
share this page if you liked it 😊
Other Related Blogs
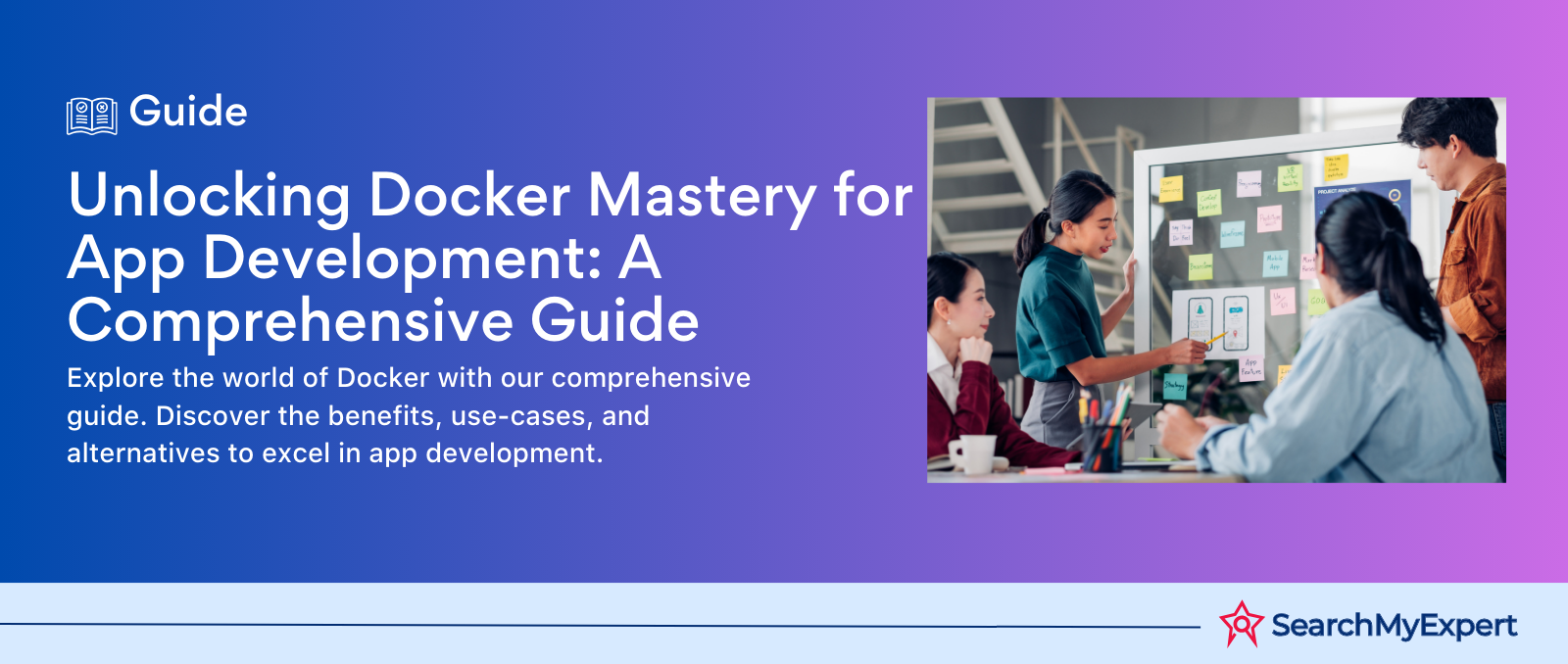
Mastering Docker for App Development: A Comprehensive Guide to Benefits, Use-Cases, and Alternatives
STAY UP TO DATE
GET PATH'S LATEST
Receive bi-weekly updates from the SME, and get a heads up on upcoming events.
Contact Us
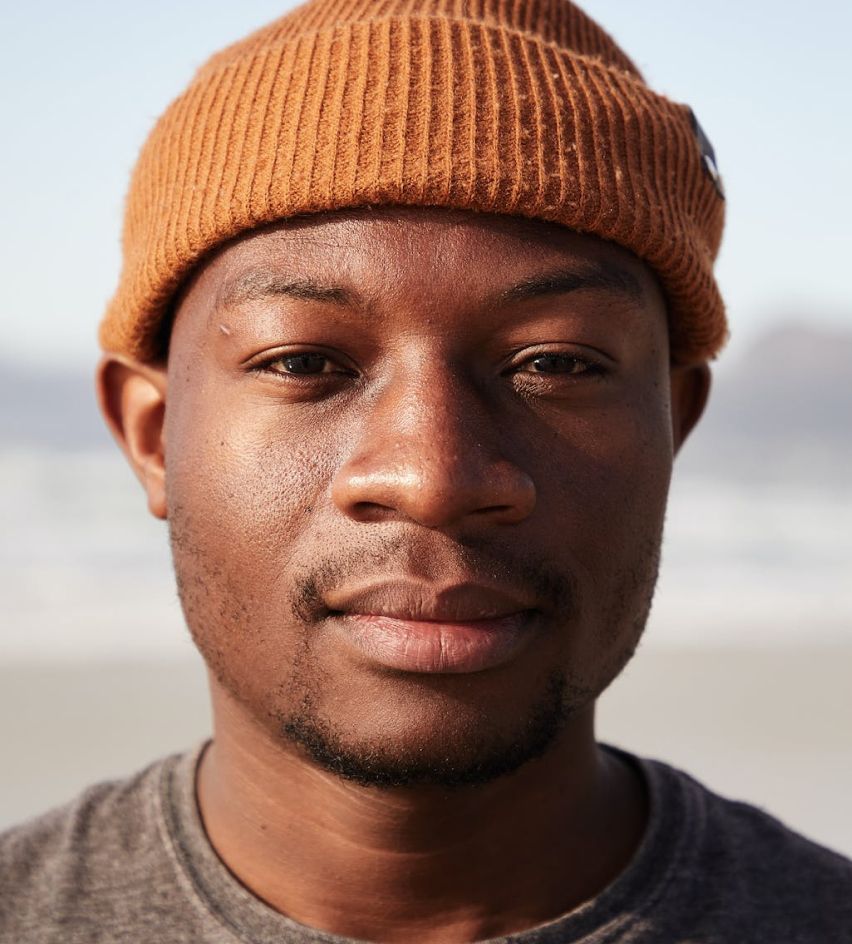
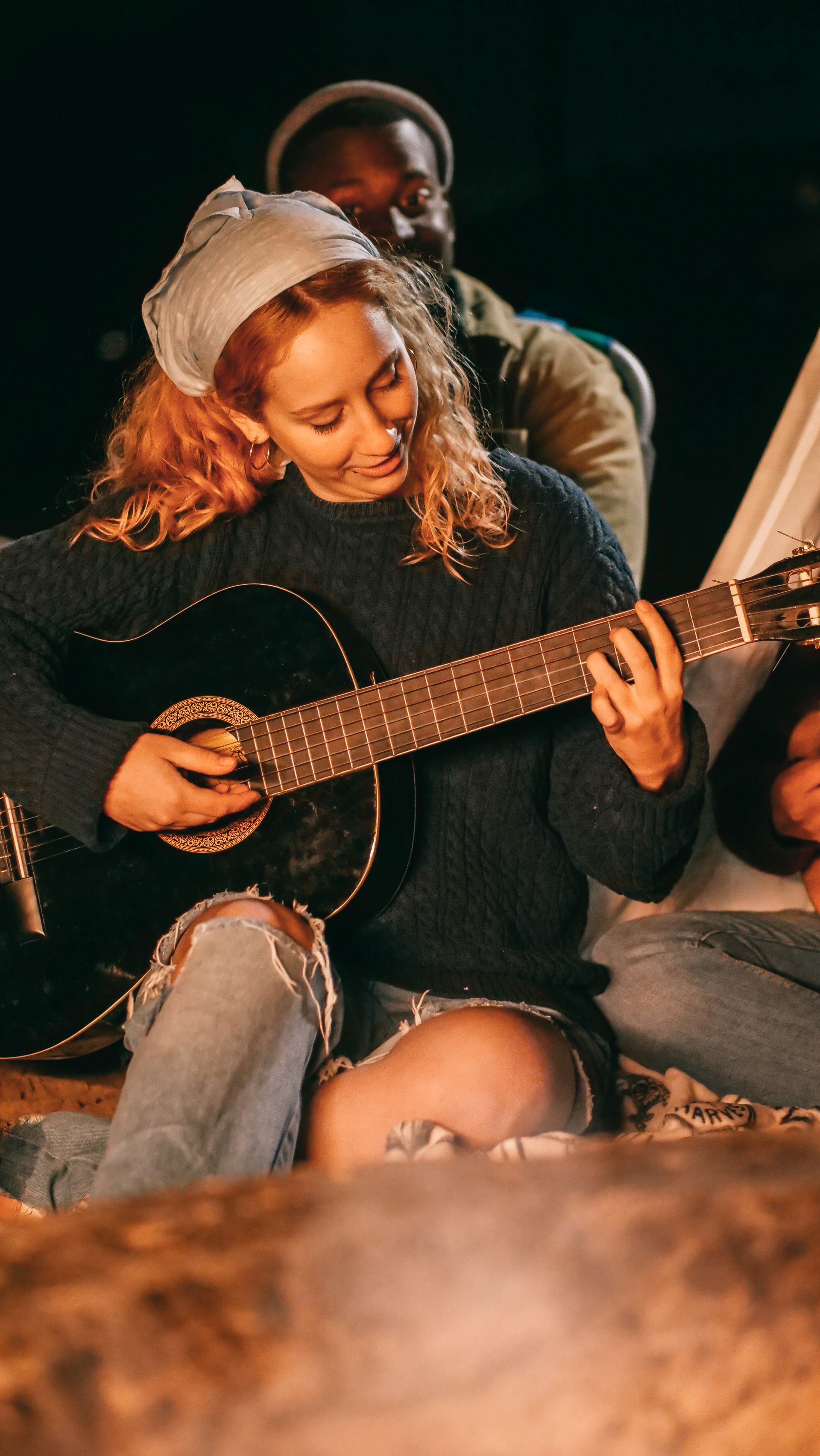