Essentials of Web App Development: A Brief Guide
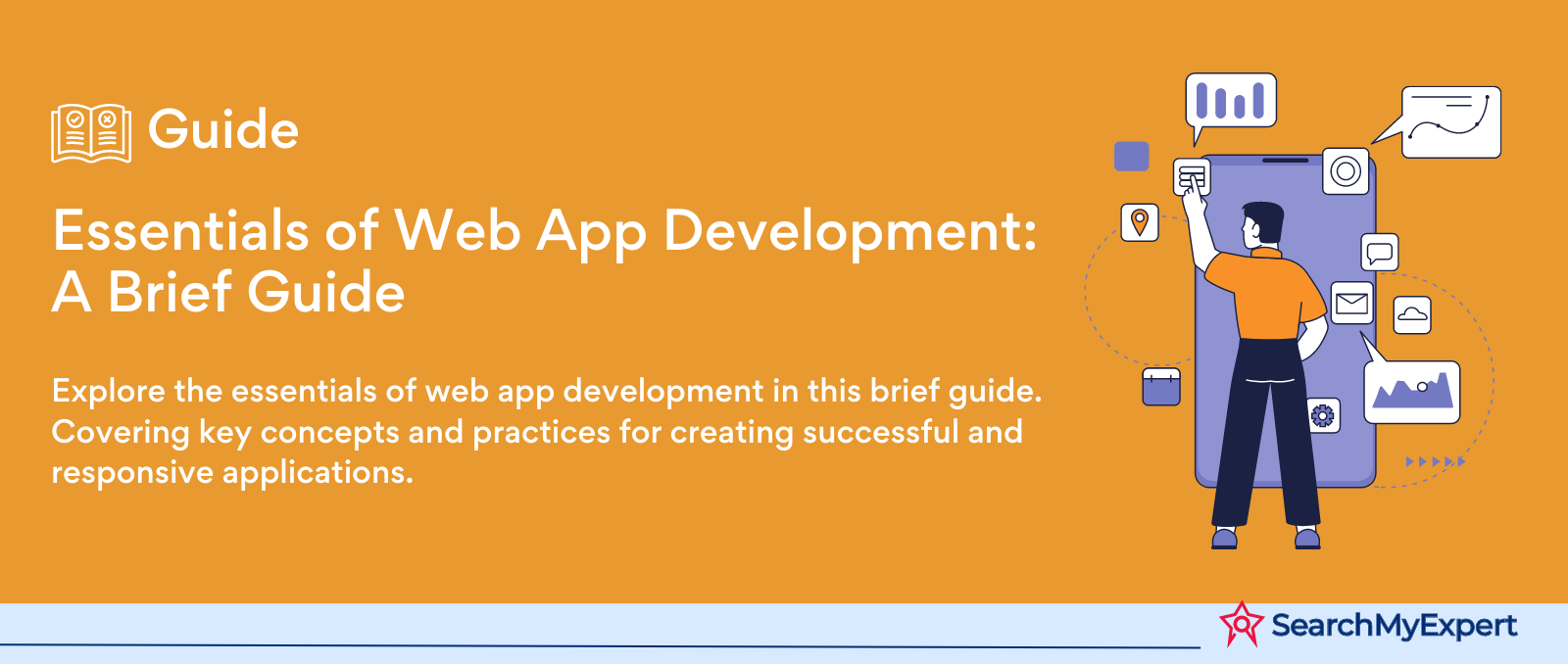
Choosing the Right Tools and Framework for Your Web Application Development
Developing a web application is an intricate process that involves a series of critical decisions. One of the most fundamental of these is selecting the appropriate tools and frameworks. This decision lays the groundwork for the development process and significantly influences the project's efficiency, scalability, and success. In this section, we will guide you through the process of understanding your project needs, exploring popular frameworks like Django and Flask, and choosing additional libraries that can enhance your web application.
Understanding Your Project Needs
Before delving into the world of frameworks and tools, it's crucial to thoroughly understand your project's requirements. The following points will help you assess the complexity, scale, and functionalities needed for your web application:
Project Scope and Complexity:
- Assess the size and complexity of your web application. Is it a simple blog or a complex e-commerce site?
- Consider the user load. Will your application need to handle a few users or thousands simultaneously?
- Evaluate the data handling requirements. Does your application need to process large amounts of data?
Required Functionalities:
- List down the functionalities your web application will offer. This includes user authentication, payment gateways, chat systems, etc.
- Determine the level of customization required for each functionality.
Future Scalability:
- Plan for the future. Consider if your application will need to scale up in terms of features or user capacity.
- Think about maintainability. How easy is it to update or modify your application?
Exploring Frameworks: Django vs Flask
Choosing the right framework is pivotal. Let's compare two popular Python frameworks: Django and Flask.
Django: The ‘Batteries-Included’ Approach:
Pros:
- Comprehensive: Offers built-in features like an ORM (Object-Relational Mapping), admin panel, and authentication support.
- Scalable: Efficiently handles high traffic and complex data operations.
- Strong Community: Offers extensive documentation and community support.
Cons:
- Steeper Learning Curve: Might be overwhelming for beginners.
- Less Flexibility: The built-in features might be excessive for simple applications.
Flask: The ‘Microframework’ Approach:
Pros:
- Lightweight and Flexible: Ideal for smaller applications and offers more control over components.
- Simplicity: Easier for beginners to grasp.
- Extensible: Easily integrates with other tools and libraries.
Cons:
- Additional Setup Required: You may need to manually add components that are automatically included in Django.
- Less Built-in Functionality: Requires more effort to implement complex features.
Selecting Additional Libraries
Beyond the core framework, you may need additional libraries for specific tasks:
- Database Management: Libraries like SQLAlchemy or Django ORM can simplify interactions with databases.
- Authentication: Consider Flask-Login or Django’s built-in authentication system for user management.
- Templating: Jinja2 is a popular choice for rendering templates in Flask, while Django has its own templating engine.
Writing Pythonic Code: A Guide to Clean and Effective Programming in Web Development
Crafting Pythonic code is a hallmark of an experienced developer. This approach not only enhances code readability and maintainability but also aligns with the best practices of Python programming. In this section, we will explore the essentials of writing Pythonic code, focusing on adhering to PEP 8 guidelines, effectively utilizing Python's data structures, and creating concise, well-documented functions.
Embracing PEP 8: Python's Style Guide
PEP 8 is more than just a style guide; it's the cornerstone of writing clean Python code. Here's how to embrace its principles:
Consistency in Naming and Formatting:
- Adopt clear and consistent naming conventions. This makes your code self-explanatory and easy to navigate.
- Ensure your code formatting aligns with PEP 8 standards. This includes proper indentation, use of white spaces, and line length management.
Readability as a Priority:
- Write code that is easy to read and understand. This not only benefits you but also others who may work on your code in the future.
- Avoid complex and cluttered code constructions. Simplicity and clarity should be your goals.
Adaptability and Flexibility:
- Be open to adapting your coding style for better readability and efficiency.
- While it's important to follow guidelines, also be flexible in situations where deviations may lead to clearer and more efficient code.
Utilizing Python's Efficient Data Structures
Python's built-in data structures are powerful tools. Using them effectively can greatly enhance the performance and organization of your code:
Lists for Ordered Collections:
- Lists are versatile and ideal for maintaining an ordered collection of items. They are particularly useful in scenarios where the order of elements matters and changes frequently.
Dictionaries for Key-Value Pairing:
- When you need fast access to elements using keys, dictionaries are the way to go. They are optimized for retrieving values when you know the key, making them essential for data mapping.
Sets for Unique Elements:
- Sets are perfect for situations where you need to ensure all elements are unique. They are highly efficient for membership testing and eliminating duplicates.
Crafting Concise and Clear Functions
The art of function writing in Python involves creating modular, reusable, and well-documented blocks of code:
Modularity and Reusability:
- Focus on breaking down complex tasks into smaller, manageable functions. This not only makes your code more readable but also allows for reusability across different parts of the application.
Clarity and Documentation:
- Each function should have a clear purpose. Avoid creating functions that perform too many tasks at once.
- Document your functions well. Even though you are not providing code examples, it's important to remember that good documentation explains the purpose and usage of the function, enhancing understanding and maintainability.
Efficient and Purpose-Driven Design:
- Design your functions to be efficient and purpose-driven. Each function should perform its intended task with minimal side effects.
Secure and Performant Development in Web Applications
In the realm of web application development, prioritizing security and performance is not just a best practice, but a necessity. This section is dedicated to guiding you through the critical aspects of secure and performant development, focusing on input validation, robust error handling, and performance optimization.
Input Validation: The First Line of Defense
Input validation is crucial for preventing security vulnerabilities and ensuring data integrity. Here’s how to effectively implement it:
Sanitize User Input:
- Treat all user input as potentially malicious. Implement stringent checks to validate the data type, format, and size of user inputs.
- Utilize regular expressions, type constraints, and length checks to validate input.
Prevent Injection Attacks:
- Be vigilant against SQL injection, XSS, and other injection attacks. Use parameterized queries and encoding techniques to neutralize harmful inputs.
- Framework-specific tools like Django’s ORM or Flask-WTF can offer additional layers of security.
Implement Robust Validation on Both Client and Server-Side:
- While client-side validation enhances the user experience, always enforce server-side validation as the main security measure.
Error Handling: Enhancing Reliability and User Experience
Effective error handling is key to maintaining the stability of your application and providing a user-friendly experience:
Graceful Error Handling:
- Ensure that your application handles errors smoothly, without exposing sensitive information or crashing.
- Implement try-except blocks to catch and handle exceptions properly.
User-Friendly Feedback:
- Provide informative and non-technical error messages to users. This helps them understand what went wrong and how to proceed.
- Log technical details for internal use, aiding in debugging and resolution.
Performance Optimization: Ensuring Speed and Efficiency
The performance of your web application directly impacts user experience and satisfaction. Here’s how to optimize it:
Identify and Address Bottlenecks:
- Regularly profile your application to identify performance bottlenecks. Tools like Python’s cProfile can be instrumental in this process.
- Focus on optimizing resource-intensive parts of your application, such as database interactions and algorithmic processing.
Implement Caching Strategies:
- Use caching to store frequently accessed data, reducing the load on databases and improving response times.
- Implement solutions like Memcached or Redis for efficient caching mechanisms.
Optimize Database Queries:
- Optimize SQL queries to minimize the response time. This includes selecting only necessary fields, using joins efficiently, and indexing critical columns.
- Regularly review and refactor your queries, especially as your application scales.
Ensuring Reliability and Functionality in Web Development
In the world of web application development, testing and debugging are pivotal processes that ensure your application functions as intended and is free from critical bugs. This section will delve into the best practices for unit testing, integration testing, and effective debugging, each playing a crucial role in building a robust and reliable web application.
Unit Testing: The Building Blocks of Reliable Code
Unit testing involves testing individual components or functions of your application. It is a key aspect of ensuring code quality and functionality:
Writing Effective Unit Tests:
- Each test should focus on a single functionality. The aim is to isolate each part of the program and show that the individual parts are correct.
- Ensure your tests are repeatable and run your tests frequently to catch new bugs as soon as they are introduced.
Coverage and Edge Cases:
- Strive for high test coverage but remember that 100% coverage doesn’t guarantee a bug-free application. Focus also on critical and complex parts of your code.
- Don’t forget to test edge cases. This includes testing for null, extreme, or unexpected inputs.
Integration Testing: Ensuring Component Harmony
While unit tests focus on individual components, integration testing ensures that different parts of your application work together seamlessly:
Testing Component Interaction:
- Focus on the interfaces between components. Ensure that data flows correctly across different parts of your application and that integrated components function together as expected.
- This is particularly important for applications with numerous modules, databases, and external services.
Simulating Real-World Scenarios:
- Create tests that simulate real user scenarios to ensure the application behaves correctly under typical usage conditions.
- Consider using automated testing frameworks to simulate a series of interactions with your application.
Debugging Effectively: Tracking Down and Fixing Bugs
Debugging is an art form. Efficiently identifying and resolving issues in your code can save significant time and resources:
Utilizing Debugging Tools and Techniques:
- Leverage debugging tools like Python’s pdb or integrated debuggers in development environments. These tools can help you step through your code, inspect variables, and understand the state of your application at any point in time.
- Logging is also a powerful tool. Effective logging can help track down when and where things go wrong.
Systematic Approach to Debugging:
- Adopt a systematic approach to debugging. Start by replicating the bug, then gradually narrow down the problematic part of the code.
- Once the issue is identified, consider the best approach to fix it. This might involve refactoring, rewriting, or sometimes even redesigning a component of your application.
Code Documentation and Readability
In the lifecycle of web application development, the importance of code documentation and readability cannot be overstated. Well-documented and readable code is not only easier to understand and maintain but also facilitates better collaboration among development teams. This section will guide you through best practices in leveraging docstrings, comments, and code formatting to achieve high standards of code documentation and readability.
Docstrings: The Foundation of Code Documentation
Docstrings play a crucial role in explaining the purpose and usage of functions, classes, and modules. Here’s how to effectively utilize them:
Writing Informative Docstrings:
- Docstrings should provide a clear explanation of what the function or class does, its parameters, return types, and any exceptions it may raise.
- Use a consistent style for docstrings throughout your codebase, such as Google style or NumPy/SciPy style.
Beyond Basics: Examples and More:
- Whenever possible, include examples in your docstrings. This helps others understand how to use the code.
- Document any peculiarities or non-obvious aspects of the code in the docstrings to provide a comprehensive understanding.
Comments: Clarifying Complex Code Sections
While docstrings explain what a function or class does, comments are used to clarify how certain parts of your code work, especially complex or non-intuitive sections:
Strategic Placement of Comments:
- Place comments near the code they are explaining. Inline comments can be used for brief explanations, while more detailed descriptions should be placed above the code block.
- Avoid stating the obvious; focus comments on why certain decisions were made or why a particular approach is used.
Clarity and Brevity:
- Comments should be clear, concise, and directly related to the adjacent code. Avoid overly verbose comments that can clutter your code.
- Remember that good code is as self-explanatory as possible. Strive to write code that is clear on its own and use comments to provide additional context where necessary.
Code Formatting: Enhancing Code Aesthetics and Readability
Consistent and logical code formatting is essential for maintaining readability:
Consistency in Style:
- Adopt a coding style guide, like PEP 8 for Python, and stick to it throughout your project. This includes guidelines on naming conventions, indentation, line length, etc.
- Use tools like linters and formatters to automatically enforce these style guidelines.
Logical Organization:
- Organize your code logically and intuitively. Group related functions and classes together and order them in a way that makes sense for someone navigating your codebase.
- Make use of whitespace and indentation to improve the readability of your code. This includes consistent use of new lines and avoiding crowded lines of code.
Managing Dependencies and Environments
In the development of web applications, managing dependencies and environments is crucial for ensuring a smooth workflow and preventing conflicts between different projects. This step-by-step guide will explore how to set up virtual environments, manage package dependencies with tools like pip and requirements.txt, and handle configuration variables securely using libraries like dotenv.
Virtual Environments: Your Isolated Workspace
Virtual environments are essential for creating isolated spaces for individual projects, which helps in managing project-specific dependencies without affecting other projects or the global Python environment.
Setting Up Virtual Environments:
- Utilize tools like Venv, included in Python 3, to create isolated environments for each project. This is crucial for avoiding version conflicts between packages used in different projects.
- Activate the virtual environment whenever you work on the project to ensure that any package installations or updates are confined to that specific environment.
Benefits of Virtual Environments:
- Maintain separate dependencies for each project, thus avoiding version conflicts.
- Replicate development environments easily, which is particularly helpful in collaborative projects and when deploying to production.
Package Management with pip and requirements.txt
Effective package management is a critical aspect of maintaining the integrity and consistency of your web application.
Using Pip for Package Installation:
- pip is a powerful tool for installing and managing Python packages. Utilize it to install packages within your virtual environment.
- Regularly update your packages to incorporate bug fixes and security patches.
requirements.txt: Tracking Dependencies:
- Maintain a requirements.txt file in your project root. This file should list all the necessary packages and their specific versions required for your project.
- Use pip freeze > requirements.txt to generate this file, ensuring that your development environment can be replicated accurately elsewhere.
Configuration Management with dotenv
Secure and efficient management of configuration settings is vital, especially when dealing with sensitive data.
Secure Storage of Sensitive Information:
- Use environment variables to store sensitive information such as API keys, database credentials, and other configuration details.
- This practice keeps sensitive data out of your source code, reducing the risk of exposing confidential information.
Implementing dotenv for Configuration Management:
- Libraries like dotenv allow you to load environment variables from a .env file at runtime.
- Keep your .env file out of version control to ensure that sensitive information remains secure. You can provide a .env.example file with dummy values as a template.
Deployment and Maintenance: Navigating the Final Stages of Web Application Development
Reaching the deployment and maintenance phase of web application development is a significant milestone. This phase entails selecting a suitable hosting provider, setting up continuous integration and delivery (CI/CD) pipelines, and implementing robust monitoring and logging systems. Each of these steps is crucial for ensuring that your application not only goes live smoothly but also remains reliable and efficient over time
Choosing a Hosting Provider: The Foundation of Your Application
The choice of a hosting provider is critical for the performance, security, and reliability of your web application.
Key Considerations for Hosting Providers:
- Security: Look for providers offering robust security features, including firewalls, SSL certificates, and regular backups.
- Performance: Consider the provider's uptime track record and server response times.
- Scalability: Ensure the provider can scale resources according to your application's growing needs.
- Support: Reliable customer support is vital, especially for resolving issues quickly and efficiently.
Popular Hosting Providers:
- Cloud services like AWS, Google Cloud, and Azure offer extensive and scalable hosting solutions.
- Traditional hosting services like Bluehost or SiteGround can also be viable options, depending on your project's needs.
Continuous Integration and Delivery (CI/CD): Streamlining Deployment
CI/CD pipelines are essential for automating the deployment process, making it faster, more consistent, and less prone to human error.
Setting Up CI/CD Pipelines:
- Implement continuous integration to automatically test your code every time you push changes, ensuring that new code doesn't break existing functionality.
- Use continuous delivery to automate the deployment of your application to the hosting environment, ensuring that your latest builds are always available to users.
Tools for CI/CD:
- Jenkins, Travis CI, and CircleCI are popular tools that offer robust CI/CD capabilities.
- Many cloud providers also offer their own CI/CD tools, such as AWS CodePipeline or Azure DevOps.
Monitoring and Logging: Keeping Your Application Healthy
Monitoring and logging are vital for maintaining the health and performance of your application post-deployment.
Implementing Monitoring Tools:
- Use monitoring tools to track your application’s performance, availability, and resource usage.
- Set up alerts for any critical issues that need immediate attention, such as downtime or performance degradation.
Effective Logging Practices:
- Implement logging to record events and transactions within your application. This is crucial for debugging issues and understanding user interactions with your app.
- Tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Splunk can be used for powerful logging and data analysis.
Conclusion:
In this comprehensive guide, we've journeyed through the critical stages of web application development, touching upon various essential aspects such as choosing the right tools and frameworks, writing clean and efficient Pythonic code, implementing secure development practices, and mastering the art of testing and debugging. We delved into the nuances of managing dependencies and environments, highlighted the importance of thorough documentation and readability, and discussed strategies for effective deployment and maintenance.
Navigate the future of software with experienced
Python Development Service Agencies.
share this page if you liked it 😊
Other Related Blogs
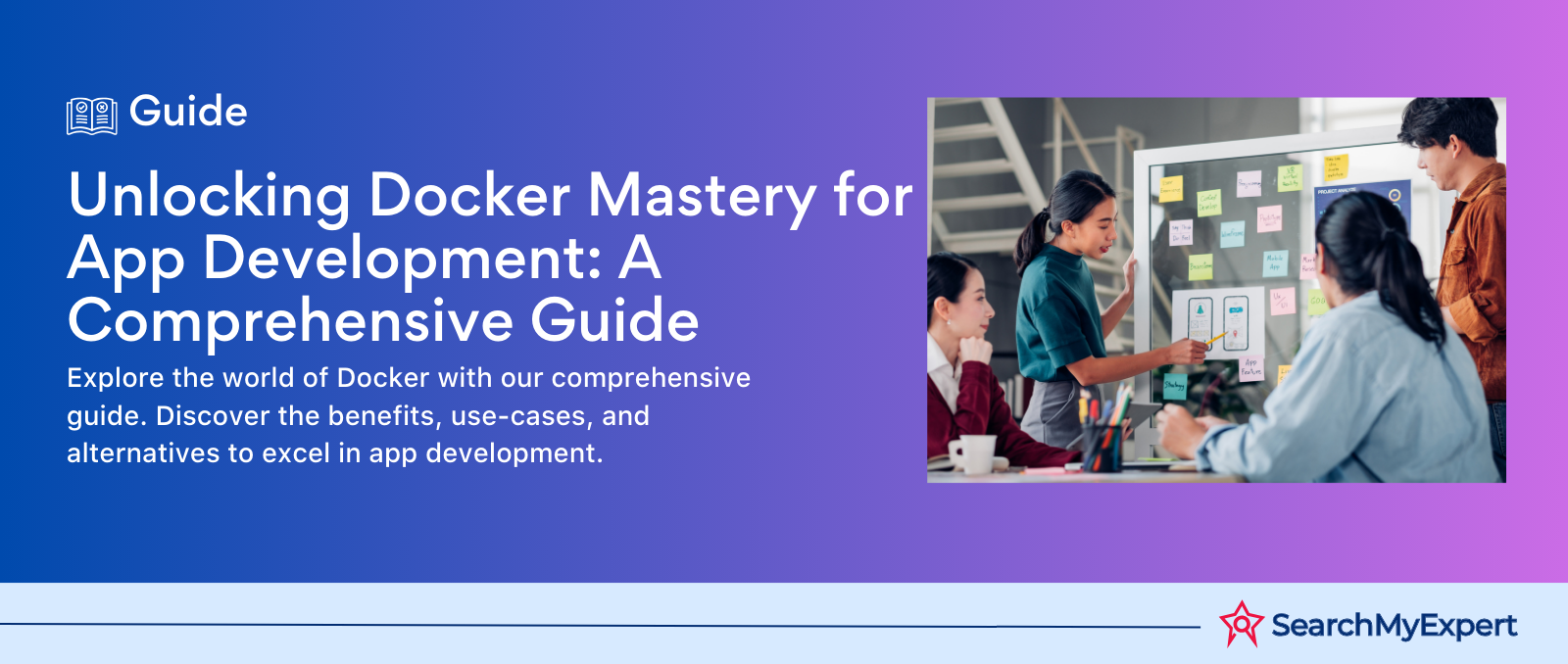
Mastering Docker for App Development: A Comprehensive Guide to Benefits, Use-Cases, and Alternatives
STAY UP TO DATE
GET PATH'S LATEST
Receive bi-weekly updates from the SME, and get a heads up on upcoming events.
Contact Us
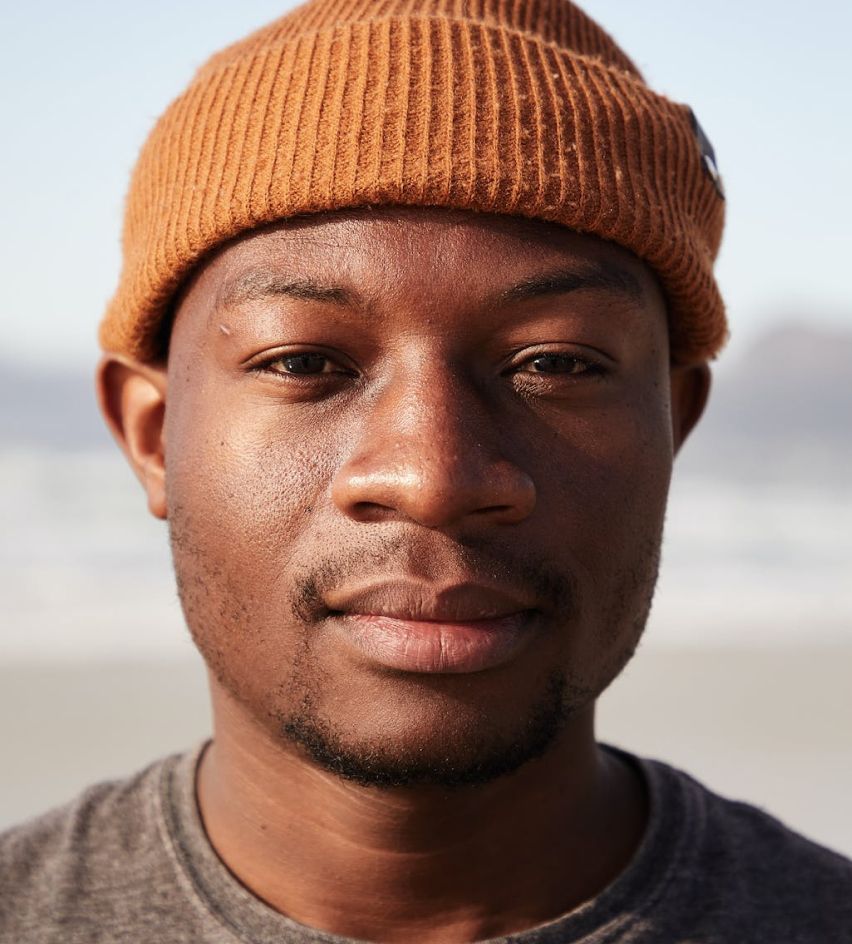
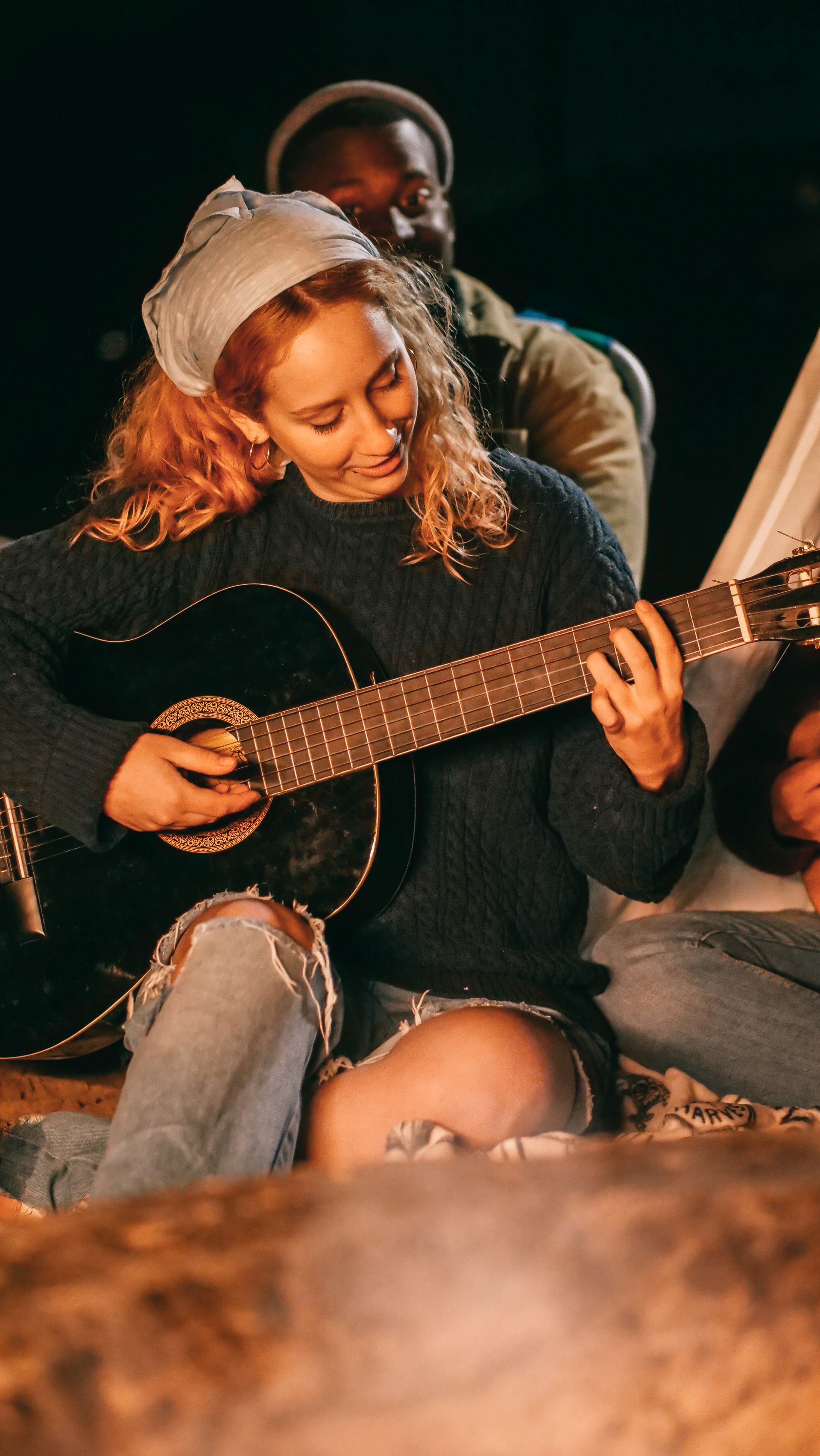